Senquip Connect App¶
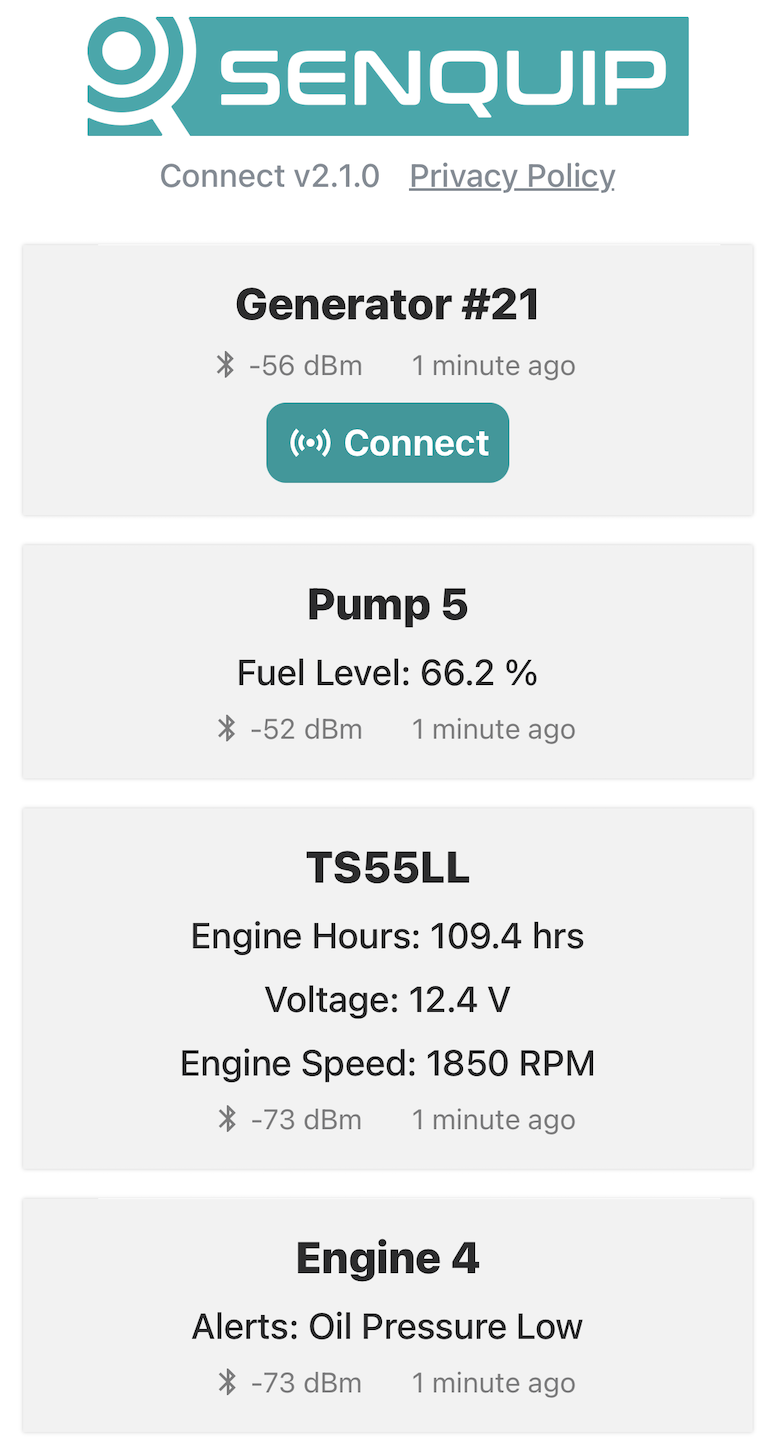
Senquip Connect App¶
The Senquip Connect app is a mobile application that allows users to connect to nearby Senquip devices using Bluetooth and view their data. The app is available for both Android and iOS devices.
Data from a Senquip device is transmitted via Bluetooth Low Energy (BLE) advertisements. The app listens for these advertisements and displays the data in a user-friendly format. The app also allows users to configure network settings on a device while in Setup Mode.
The amount of data that can be transmitted via BLE advertisements to the Senquip app is limited to 27 bytes. Due to this limitation, the Senquip Connect app uses a custom format to interpret and display common information such as engine hours, fuel level, voltage etc. The app also allows for custom text to be sent and displayed.
Warning
Some mobile devices may cache BLE advertising data and not display the latest infomation. To ensure the latest data is displayed, it is recommended to close and re-open the app or turn off and on Bluetooth on the mobile device.
Pre-defined Data Types¶
There are two methods to encode data:
As a string. The data type code should appear first, followed by the ASCII string. For example, to send the text ‘Device Name’ as a string, use the format
"\x80" + "Device Name"
.As a floating point number using SQ.encode(value,SQ.FLOAT). The data type code should appear first, followed by the SQ.encode(value,SQ.FLOAT). An optional unit string can then appear after the encoded value. For example, to send the float value 123.456 as an engine hours value with ‘hrs’ as the unit, use the format
"\x90" + SQ.encode(123.456,SQ.FLOAT) + "hrs"
.
An encoded float value will use 4 bytes from the available data payload. Plus 1 byte for the type.
The following data types are supported by the Senquip Connect app:
TITLE_FORMAT_ID = "\x80"
- Text for the title of the entry that appears on the Senquip Connect app. Typically used to send the device name.MEASUREMENT_FORMAT_ID = "\x81"
- Text for a measurement line.ENGINE_HOURS_FORMAT_ID = "\x90"
- Engine hours as a float.MOVEMENT_HOURS_FORMAT_ID = "\x91"
- Movement hours as a float.VIBRATION_HOURS_FORMAT_ID = "\x92"
- Vibration hours as a float.IGNITION_HOURS_FORMAT_ID = "\x93"
- Ignition hours as a float.ENGINE_SPEED_FORMAT_ID = "\x94"
- Engine speed as a float.FUEL_LEVEL_FORMAT_ID = "\x95"
- Fuel level as a float.FLOW_RATE_FORMAT_ID = "\x96"
- Flow rate as a float.LEVEL_FORMAT_ID = "\x97"
- Level as a float.VOLTAGE_FORMAT_ID = "\x98"
- Voltage as a float.CURRENT_FORMAT_ID = "\x99"
- Current as a float.STATE_FORMAT_ID = "\x9a"
- State as a string.ALERTS_FORMAT_ID = "\x9b"
- Alerts as a string.
Other data types not listed can be added to the app by request. Contact Senquip for more information.
Note
Only 2 decimal places are displayed for float values. Please convert to appropriate units before sending to the app.
If the total length of the data exceeds 27 bytes, the data will be truncated and parts may not appear in the app.
Custom Text Example¶
This example shows how to send data to the Senquip Connect app using the custom TITLE and MEASUREMENT text formats.
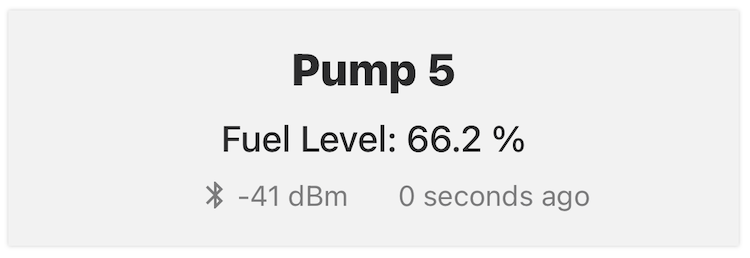
Example Output in Senquip Connect app for code shown below¶
load('senquip.js');
SQ.set_data_handler(function(data)
{
let TITLE_FORMAT_ID = "\x80";
let MEASUREMENT_FORMAT_ID = "\x81";
let fuel_level = 66.2365;
let device_name = "Pump 5";
let ble_data = MEASUREMENT_FORMAT_ID + "Fuel Level: " + SQ.to_fixed(fuel_level, 1) + " %" + TITLE_FORMAT_ID + device_name;
BLE.sq_adv(ble_data);
}
Parameters Encoded as Floats Example¶
This example shows how to send data to the Senquip Connect app by encoding parameters as a float and adding a unit string.
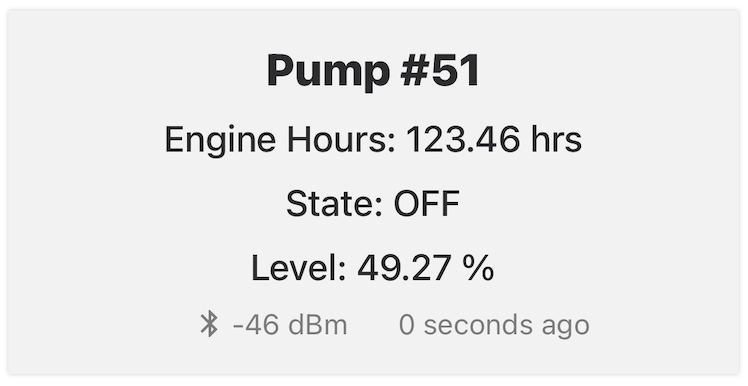
Example Output in Senquip Connect app for code shown below¶
load('senquip.js');
load('api_config.js');
SQ.set_data_handler(function(data)
{
let device_name = Cfg.get('device.name'); // "Pump #515"
let engine_hrs = 123.456;
let state_str = "OFF";
let level = 49.273;
let ble_data = "\x90" + SQ.encode(engine_hrs,SQ.FLOAT) + "hrs\x9a" + state_str + "\x97" + SQ.encode(level,SQ.FLOAT) + "%" + "\x80" + device_name;
BLE.sq_adv(ble_data);
}
Note
The length of ble_data in this example exceeded 27 bytes, therefore the last character of the device name is missing.